How to Build a Simple Application Powered by ChatGPT
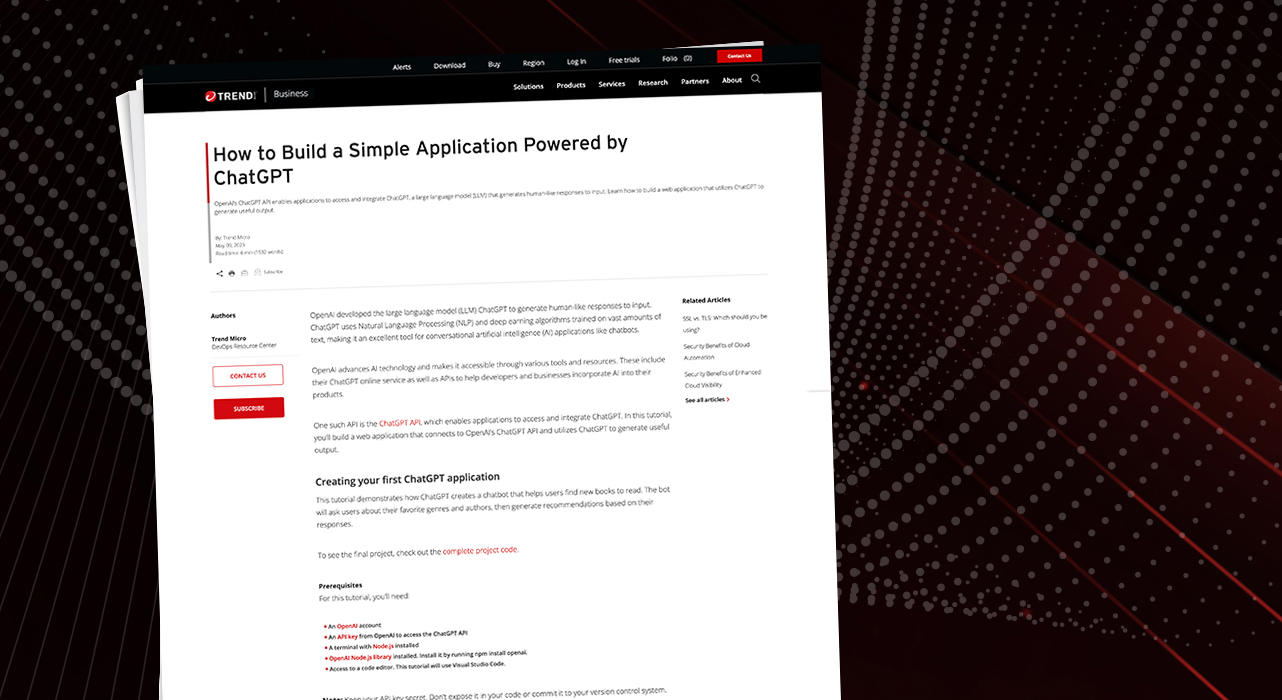
OpenAI developed the large language model (LLM) ChatGPT to generate human-like responses to input. ChatGPT uses Natural Language Processing (NLP) and deep earning algorithms trained on vast amounts of text, making it an excellent tool for conversational artificial intelligence (AI) applications like chatbots.
OpenAI advances AI technology and makes it accessible through various tools and resources. These include their ChatGPT online service as well as APIs to help developers and businesses incorporate AI into their products.
One such API is the ChatGPT API, which enables applications to access and integrate ChatGPT. In this tutorial, you’ll build a web application that connects to OpenAI’s ChatGPT API and utilizes ChatGPT to generate useful output.
Creating your first ChatGPT application
This tutorial demonstrates how ChatGPT creates a chatbot that helps users find new books to read. The bot will ask users about their favorite genres and authors, then generate recommendations based on their responses.
To see the final project, check out the complete project code.
For this tutorial, you’ll need:
- An OpenAI account
- An API key from OpenAI to access the ChatGPT API
- A terminal with Node.js installed
- OpenAI Node.js library installed. Install it by running npm install openai.
- Access to a code editor. This tutorial will use Visual Studio Code.
Note: Keep your API key secret. Don’t expose it in your code or commit it to your version control system.
OpenAI offers a free trial allowing developers to test the ChatGPT API without incurring charges. After this trial period ends, you must sign up for a paid plan to continue using the service.
The ChatGPT API typically sends a message to the API and receives a response back. To generate a response, you must pass the message along with some configuration options to the openai.createChatCompletion method.
This is an example “hello world” application demonstrating the different options:
const { Configuration, OpenAIApi } = require(“openai”);
const configuration = new Configuration({
organization: process.env.ORG,
apiKey: process.env.API_KEY,
});
const openai = new OpenAIApi(configuration);
const getResponse = async() => {
const response = await openai.createChatCompletion({
model: “gpt-3.5-turbo”,
max_tokens: 30
messages: [{role: “user”, content: “Hello world”}]
}).catch((err)=>console.log(err.response));
console.log(response)
}
getResponse()
Here’s a description of the options you passed to the openai.createCompletion method:
- model: The ID of the GPT-3 engine to use
- max_tokens: The maximum number of tokens to use in the response
- messages: The messages the API must create responses for. The ChatGPT API introduction has a full explanation of the role property provided with the message content.
Refer to the OpenAI API documentation for details about all available options.
To create a new Node.js application, open your terminal or command prompt. First, create a new directory called recommend_a_book.
Navigate into the directory and run the following command:
npm init –y
This initializes a default Node.js app.
First, create a simple command line app to allow users to interact with the chatbot. Use the readline module in Node.js to accept input from the user and display output from the ChatGPT API.
Set the API key created earlier as an environment variable. Enter the following command in your terminal:
export API_KEY='<insert API key here>’
Now, replace <insert API key here> with your OpenAI API key.
Add the code for this application to a file called index.js in the root of your project directory. The final index.js should appear as follows:
const { Configuration, OpenAIApi } = require(“openai”);
const readline = require(‘readline’);
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
const configuration = new Configuration({
apiKey: process.env.API_KEY,
});
const openai = new OpenAIApi(configuration);
const getResponse = async(genres, authors) => {
const response = await openai.createChatCompletion({
model: “gpt-3.5-turbo”,
max_tokens: 150,
messages: [{role: “user”, content: `recommend some books for someone who loves the following genres: “${genres}” and the following authors: “${authors}”`}]
}).catch((err)=>console.log(err.response));
return response.data.choices[0].message.content
}
const getGenres = () => {
return new Promise((resolve, reject) => {
rl.question(‘List your favourite genres with a comma after each (e.g. sci-fi, fantasy..):\n’, (answer) => {
resolve(answer)
})})
}
const getAuthors = () => {
return new Promise((resolve, reject) => {
rl.question(‘List your favourite authors with a comma after each (e.g. phillip k dick, george rr martin..):\n’, (answer) => {
resolve(answer)
})
})
}
const run = async() => {
const genres = await getGenres()
const authors = await getAuthors()
rl.close()
console.log(await getResponse(genres, authors))
}
run()
The application asks the user to provide a list of their favorite genres and authors through the getGenres and getAuthors functions. You can then capture their input from the command line and send it to the ChatGPT API:
Read More HERE